prisma学习使用
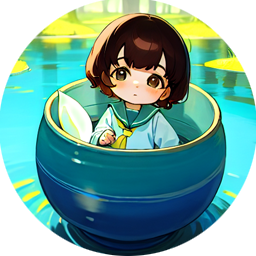
prisma
nodejs orm工具
依赖:prisma
(-D)
Installation
- 安装依赖:
npm install prisma -D
- 初始化:
npx prisma init --datasource-provider sqllite
- 建模,文件:schema.prisma
1 | model User { |
npx prisma migrate dev --name init
:三个作用:创建sql文件;更新数据库内容;生成ClientAPI。/ 也可以使用npx prisma generate生成ClientAPI。- 连接数据库
1 | import { PrismaClient } from '@prisma/client' |
Create/Read 插入/读取
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30// create
//单个创建
const user = await prisma.user.create({
data: {
name: 'Alice',
email: '[email protected]',
},
})
// 嵌套创建
const user = await prisma.user.create({
data: {
name: 'Bob',
email: '[email protected]',
posts: {
create: [
{
title: 'Hello World',
published: true
},
{
title: 'My second post',
content: 'This is still a draft'
}
],
},
},
})
console.log(user)1
2
3
4
5
6
7
8
9
10
11// read
const users = await prisma.user.findMany()
console.log(users)
// 嵌套查询
const usersWithPosts = await prisma.user.findMany({
include: {
posts: true,
},
})
console.dir(usersWithPosts, { depth: null })Prisma的工具:Prisma Studio
1
npx prisma studio
Usage
Database
schema与数据库同步
prisma支持从schema更新数据库结构,使用migrate子参数。
prisma会生成“迁移文件”记录每次数据库结构发生的变化。
prisma的migrate有两种方式:
- dev:开发环境模式。
- 生成迁移文件并应用到数据库;
- 可选支持seed脚本,seed脚本可以通过typescript脚本为数据库添加随机数据。注:==配置该功能每次进行migrate就会清理数据库所有数据==。==不配置不会清理数据库数据==。==可以通过–skip-seed跳过==。
- deploy:生产环境模式。
- 不生成迁移文件,将最后一次的迁移文件应用到数据库。
- 不会清理数据库。
1 | npx prisma migrate dev/deploy [--skip-seed] [--name 迁移记录名称] |
Nodejs: CRUD
CRUD,即增查改删。
Create
基础
1 | const newUser = await prisma.user.create({ |
扩展
1 | const newUser = await prisma.user.create({ |
1 | //插入多条数据 |
Read
基础
1 | // 查询所有数据 |
扩展
1 | const users = await prisma.user.findMany({ |
1 | // where |
Update
1 | // 单条数据更新 (这里要让where只匹配到一个数据) |
Delete
1 | await prisma.user.delete({where: {...}}); |
Schema
基础
1 | model User { |
扩展
1 | model User { |
参数类型:Int, String, Boolean, DateTime, Float, Json, Bytes
定义字段在数据库的字段类型: @db.TYPE。比如:@db.Int
- Int, VarChar(255), Text, Boolean, Timestamp, Decimal(precision, scale)
1 | # 一对多 |
OTHER
从数据库生成schema
1 | npx prisma db pull |
新特性:通过schema分离
被删除的部分
1 | password String @notNull # 验证:非空 |
被误导了,没有这一部分。
评论