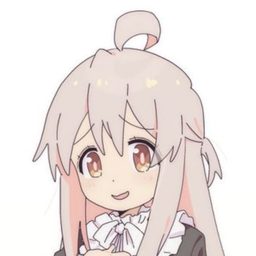
SpringBoot的异步任务
使用
在需要异步执行的方法上面添加注解@Async
1
2
3
4
5
6//告诉Sping这是一个异步的方法
public void hello() throws InterruptedException {
Thread.sleep(3000);
System.out.println("数据正在处理...");
}在主方法的类上面添加注解@EnableAsync
1
2
3
4
5
6
7//开启异步任务功能
public class SpringBootStudy4TaskApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootStudy4TaskApplication.class, args);
}
}
表现:
创建一个Controller调用Service的hello()方法;
1
2
3
4
5
6
7
8
9
10
11
public class AsyncController {
AsyncService service;
public String hello() throws InterruptedException {
service.hello();
return "OK";
}
}在网页加载过程中,没有等待3000毫秒,直接加载出OK;
3000毫秒后,日志输出“数据正在处理…”。
service.hello()执行过程没有阻塞线程,而是在新线程中运行。
SpringBoot发送邮件
步骤
在application.properties下配置相关配置
1
2
3spring.mail.username=[email protected]
spring.mail.password=xxxxxxxxxxxxxxx
spring.mail.host=smtp.qq.comusername为邮箱名,password为邮件服务器给的密钥,host为邮件服务器的smtp域名。
发送邮件
1
2
3
4
5
6
7
8
9
10
11
JavaMailSenderImpl mailSender;//创建一个JavaMailSenderImpl引用并使用@Autowired注解
void contextLoads() {
SimpleMailMessage mailMessage = new SimpleMailMessage();
mailMessage.setSubject("这也是一个标题");
mailMessage.setText("这个是一个文本这个是一个文本这个是一个文本这个是一个文本这个是一个文本");
mailMessage.setTo("[email protected]");
mailMessage.setFrom("[email protected]");
mailSender.send(mailMessage);
}创建一个JavaMailSenderImpl引用并使用@Autowired注解;
使用SimpleMailMessage新建一个邮件对象,设定邮件的Subject、Text、来源和去向,然后使用send方法将邮件发出;
发送邮件2
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
void contextLoads2() throws MessagingException {
//一个复杂的邮件
MimeMessage mimeMessage = mailSender.createMimeMessage();
//组装
MimeMessageHelper helper = new MimeMessageHelper(mimeMessage,true,"utf-8");
helper.setSubject("这个又又是一个标题");
helper.setText("<p style='color:red'>这是一个测试学习用的邮件</p>",true);
//附件
helper.addAttachment("1.jpg",new File("D:/Document/pictures/youji.jpg"));
helper.addAttachment("2.gif",new File("D:/Document/pictures/t.gif"));
//来源 目的邮箱
helper.setTo("目的邮箱");
helper.setFrom("来源邮箱");
//发送
mailSender.send(mimeMessage);
}这是一个创建复杂邮件的过程,相对简单邮件,这里可以添加内容为html内容、添加附件等;
创建一个MimeMessage对象,然后使用MimeMessageHelper包装MimeMessage对象,使用MimeMessageHelper对象对邮件进行一些配置,最后将邮件发出。
定时执行任务
步骤
在主程序添加@EnableScheduling定时任务功能注解;
1
2
3
4
5
6
7//开启定时任务功能的注解
public class SpringBootStudy4TaskApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootStudy4TaskApplication.class, args);
}
}在需要定时执行的任务上方添加@Scheduled注解;
1
2
3
4
5//在一个特定的时间执行这个方法
public void hello(){
System.out.println("hello,执行一个定时任务..中!");
}在时间到达cron表达式指向的时间时,就会触发执行这个方法。
Cron表达式
简单示例
1 | 0 * * * * 0-7 |
表示在 星期1-7 每月 每日 每时 每分 0秒 这个时间触发一次。
(也就是 每到一个秒数为0的时间,触发一次)
基本格式
通过Cron表达式可以实现指定一个、一段、一些时间去触发某些任务;
1 | 秒 分 时 日 月 周 |
通过一个空格隔开,分别表示一个单位,每个单位通过一个简易的表达式表现在哪些时间执行任务。
单个格式
10/5
,从10开始,每过5个单位触发一次;30/5 0 0 * * ?
每天的0时0分,从30秒开始,每过5秒执行一次;0 0/5 * * * ?
每过5分钟执行一次;
10-30
,表示在10-30区间;10,20
,表示10和20两个值;*
,表示任意值;?
,只能用在日和周上,因为日和周会互相影响,若要取消影响,只需在不需要的属性上添加“?”。如:0 0 0 1 * ?
表示每月的1日0点触发;0 0 0 ? * 3
表示每周三的0点触发;0 0 0 * * ?
每天的0点触发。
6#3
,只能用在周上,表示第三个星期五。0 0 0 ? * 6#3
,每月的第三个星期五的0点触发。
L
,可以放在日和周上,- 日上表示最后一天;
0 0 0 L * ?
表示每月的最后一天的0点;
- 周上表示最后周几,使用”数字L”表达;
0 0 0 ? * 5L
表示每月的最后一个星期四的0点;
- L不要和
- 或 ,
一起使用,否则会出现意想不到的结果。
- 日上表示最后一天;
3W
,放在日上,表示距离3号最近的工作日;LW
,放在日上,表示最后一个工作日。
范围
域 | 字段 | 取值 | 特殊字符 | 是否必须 | 备注 |
---|---|---|---|---|---|
秒 | Seconds | 0-59 | , - * / | 是 | |
分 | Minutes | 0-59 | , - * / | 是 | |
时 | Hours | 0-23 | , - * / | 是 | |
日 | DayofMonth | 1-31 | , - * / ? L W | 是 | |
月 | Month | 1-12 或 JAN-DEC | , - * / | 是 | |
星期 | DayofWeek | 1-7 或 SUN-SAT | , - * / ? L # | 是 | 日 1 2 3 4 5 6 |
年 | Year | 1980-2099 | , - * / | 否 |