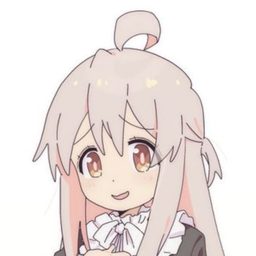
SpringBoot如何启动
入口
1 | //标注这个类是一个SpringBoot应用 |
SpringApplication.run(SpringBootForthProjectApplication.class, args);
- 推断应用的类型是普通的项目还是Web项目;
- 查找并加载所有可用初始化器,设置到initializers属性中;
- 找出所有的应用程序监听器,设置到listeners属性中;
- 推断并设置main方法的定义类,找到运行的主类;
SpringBoot全局配置文件
默认文件名
两种方案:
- application.properties
- 语法结构:key=value
- application.yml
- 语法结构:key:空格value
作用:修改SpringBoot自动配置的默认值。
文件位置
可以在以下几个位置放置配置文件:
- file:./config/ 项目目录下config文件夹中
- file:./ 项目根目录下
- classpath:/config/ 资源目录的config目录下
- classpath:/ 资源目录根目录下
优先级顺序也按照上面的顺序来。
不同环境下的配置文件
有三种环境:默认、dev、test。(默认、开发、测试)
多文件方式
创建三个文件,其中没有后缀表示默认,dev表示开发,test表示测试;
在application.yaml添加spring.profiles=指定使用的配置环境,
1
2
3spring:
profiles:
active: dev示例中表示使用dev环境。
配置的环境名可以自定义
单文件方式
在application.yaml中添加以下内容
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22server:
port: 8081
spring:
profiles:
active: dev
server:
port: 8082
spring:
config:
activate:
on-profile: dev
server:
port: 8083
spring:
config:
activate:
on-profile: test使用”—“分隔开三种配置。在示例中,第一部分为默认的配置,spring.profiles.active指定现在使用的配置。第二部分为dev配置,第三部分为test配置。
- 在默认配置中使用spring.profiles.active指定要使用的配置环境
- spring.config.activate.on-profile声明当前位置的配置名
使用即可。
yaml语法
1 | # 普通的key-value |
Spring的@Autowired和@Value实现对象自动初始化
创建一个Dog类,放入无参构造方法、getter和setter,对类使用@Component注解。对需要赋值的变量使用@Value注解,值为要赋的值。
1
2
3
4
5
6
7
8
9
10//将类加入Spring底层
public class Dog {
//自动装载的默认值
private String name;
//这里只能写字符串,整数也要用引号括住
private Integer age;
//toString方法、getter方法、setter方法、无参构造方法、有参构造方法这里省略
}在要使用的类中,创建一个引用,使用@Autowired注解,即可实现自动初始化;
1
2
3
4
5
6
7
8
9
10
11
class SpringBootForthProjectApplicationTests {
private Dog dog;
void contextLoads() {
System.out.println(dog);
}
}写一个测试类,测试结果。
1
Dog{name='张三', age=12}
通过yaml实现对象自动初始化
- 在application.yaml中添加以下内容:
1 | person: |
- 创建一个Person类,添加注解
@ConfigurationProperties(prefix = "person")
,其中prefix前缀的值为application.yaml配置的对应的对象的名,也就是person。
1 |
|
- 运行测试
1 |
|
1 | Person{name='zhangsan', age=3, happy=false, birth=Fri Sep 09 00:00:00 CST 2022, maps={k1=v1, k2=v2}, lists=[code, girl, zhangsan], dog=Dog{name='lisi', age=3}} |
注意:
@configurationProperties使用后会标红,解决办法:
在pom.xml中添加:
1
2
3
4
5<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>重启idea。
这个依赖的作用是:添加依赖后,可以用@ConfigurationProperties注释的项轻松生成自己的配置元数据文件,在编写application.yml文件可以有相关提示。
部分SPEL表达式
${random.uuid}
随机
random.uuid:获得一个随机的UUID
random.int:获得一个整数
示例1:${person.hello:Lisi}张三
在对象person中寻找hello,若有hello则用hello的值,没有则用“Lisi”代替,然后拼接“张三”。
代码示例
1 | person: |
运行结果:
1 | Person{name='zhangsand4c877dd-b714-468f-b6f9-c4a4b899a8d0', age=-161320060, happy=false, birth=Fri Sep 09 00:00:00 CST 2022, maps={k1=v1, k2=v2}, lists=[code, girl, zhangsan], dog=Dog{name='张三_LiSi', age=3}} |
松散绑定
在使用yaml实现对象自动赋值过程中,yaml中对象名和实际对象名中,驼峰命名和带“-”的命名可以对应起来。如:firstName和first-name。(properties不支持)
示例
dog类:
1 |
|
yaml中的dog:
1 | dog: |
运行结果:
1 | Dog{firstName='Qwert', age=6} |
JSR303校验
@Validated
JSR303校验指,Spring提供注解可以直接校验变量的值。
在变量前添加指定注解,可以实现注解对应的功能。
依赖
1 | <dependency> |
使用
- 在类前添加@Validated注解;
- 在需要检查的对象前添加相应的校验注解。
包
位于jakarta.validation下的javax.validation.constraint里。
代码示例
在yml文件中
1 | student: |
一个student类
1 |
|
运行测试
1 |
|
1 | Student{id=3, name='Obsidian', email='[email protected]'} |
可以使用的注解
空检查
注解名 | 介绍 |
---|---|
@Null | 验证对象是否为空。 |
@NotNull | 验证对象不为空。不检查长度为0的字符串。 |
@NotBlank | 验证约束字符串不是null,且字符串trim后长度大于0。(trim:去掉字符串前后空格) |
@NotEmpty | 验证约束元素是否为空或null。 |
Boolean检查
注解名 | 介绍 |
---|---|
@AssertTrue | 验证Boolean对象是否为true |
@AssertFalse | 验证Boolean对象是否为false |
长度检查
注解名 | 介绍 |
---|---|
@Size(min=,max=) | 验证对象(Array、Collection、Map、String)长度是否在给定范围内 |
@Length(min=,max=) | 验证被注解的字符串的长度是否在给定范围内 |
日期检查
注解名 | 介绍 |
---|---|
@Past | 验证Date和Calendar对象是否在当前时间之前 |
@Future | 验证Date和Calendar对象是否在当前时间之后 |
字符串检查
注解名 | 介绍 |
---|---|
@Pattern | 验证String对象是否符合正则表达式的规则 |
其他
使用properties配置文件
类前用@PropertySource注解
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
public class Person_properties {
private String name;
private Integer age;
private Boolean happy;
private Date birth;
private Map<String,Object> maps;
private List<Object> lists;
private Dog dog;
//Contsructor、getter、setter、toString
}在application.yaml文件中
1
2
3
4person2.name=zhangsan
person2.birth=2020/2/2
person2.age=123
person2.happy=false运行测试
1
2
3
4
5
6
7
8
9
10
11
class SpringBootForthProjectApplicationTests {
private Person_properties person;
void contextLoads() {
System.out.println(person);
}
}1
Person2{name='zhangsan', age=123, happy=false, birth=Sun Feb 02 00:00:00 CST 2020, maps=null, lists=null, dog=null}
修改的内容
单文件方式配置SpringBoot多环境
旧版本中
使用spring.profiles声明当前使用的配置名。
新版本中
使用spring.config.activate.on-profile声明当前使用的配置名。